class: center, middle, inverse, title-slide # Programming with data ## Writing functions ### Ben Baumer ### SDS 192Oct 19, 2020(
http://beanumber.github.io/sds192/lectures/mdsr_scale_01-functions.html
) --- ## Functions Do you ever find yourself with `.Rmd` files that look like this? ```r my_df1 %>% ... # Do some stuff to my_df1 ... my_df2 %>% ... # Do the same stuff to my_df2 ... my_df3 %>% ... # And again to my_df3 ... ``` --- ## For example What if I want to draw the same kind of plot several times? ```r my_df1 %>% ggplot(aes(x = var1, y = var2, color = var3)) + geom_point() + geom_line() my_df2 %>% ggplot(aes(x = varA, y = varB, color = varC)) + geom_point() + geom_line() my_df3 %>% ggplot(aes(x = var1A, y = var2B, color = var3C)) + geom_point() + geom_line() ``` --- background-image: url(http://www.science.smith.edu/~jcrouser/SDS192/recipe.png) background-size: contain --- ## User-defined functions ```r name_of_function <- function(data, var = "value") { ... ... <valid R code> ... ... return(x) } ``` - inputs: - the *arguments*: `data`, `var` - `data` is required - `var` is optional -- has a default value of `"value"` - output: - the *return* value - by default output of last line in function - here, explicitly the object `x` --- ## Scoping: global variables OK ```r my_cars <- function(mod, n = 6) { * mpg %>% filter(model == mod) %>% head(n) } my_cars("civic") ``` ``` ## # A tibble: 6 x 11 ## manufacturer model displ year cyl trans drv cty hwy fl class ## <chr> <chr> <dbl> <int> <int> <chr> <chr> <int> <int> <chr> <chr> ## 1 honda civic 1.6 1999 4 manual(… f 28 33 r subcomp… ## 2 honda civic 1.6 1999 4 auto(l4) f 24 32 r subcomp… ## 3 honda civic 1.6 1999 4 manual(… f 25 32 r subcomp… ## 4 honda civic 1.6 1999 4 manual(… f 23 29 p subcomp… ## 5 honda civic 1.6 1999 4 auto(l4) f 24 32 r subcomp… ## 6 honda civic 1.8 2008 4 manual(… f 26 34 r subcomp… ``` --- ## Default values ```r *my_cars <- function(mod = "civic", n = 6) { mpg %>% filter(model == mod) %>% head(n) } *my_cars() ``` ``` ## # A tibble: 6 x 11 ## manufacturer model displ year cyl trans drv cty hwy fl class ## <chr> <chr> <dbl> <int> <int> <chr> <chr> <int> <int> <chr> <chr> ## 1 honda civic 1.6 1999 4 manual(… f 28 33 r subcomp… ## 2 honda civic 1.6 1999 4 auto(l4) f 24 32 r subcomp… ## 3 honda civic 1.6 1999 4 manual(… f 25 32 r subcomp… ## 4 honda civic 1.6 1999 4 manual(… f 23 29 p subcomp… ## 5 honda civic 1.6 1999 4 auto(l4) f 24 32 r subcomp… ## 6 honda civic 1.8 2008 4 manual(… f 26 34 r subcomp… ``` --- ## Default values overridden ```r my_cars("jetta", n = 3) ``` ``` ## # A tibble: 3 x 11 ## manufacturer model displ year cyl trans drv cty hwy fl class ## <chr> <chr> <dbl> <int> <int> <chr> <chr> <int> <int> <chr> <chr> ## 1 volkswagen jetta 1.9 1999 4 manual(m5) f 33 44 d compa… ## 2 volkswagen jetta 2 1999 4 manual(m5) f 21 29 r compa… ## 3 volkswagen jetta 2 1999 4 auto(l4) f 19 26 r compa… ``` --- ## Naming arguments optional ```r my_cars("camry", 2) ``` ``` ## # A tibble: 2 x 11 ## manufacturer model displ year cyl trans drv cty hwy fl class ## <chr> <chr> <dbl> <int> <int> <chr> <chr> <int> <int> <chr> <chr> ## 1 toyota camry 2.2 1999 4 manual(m5) f 21 29 r midsi… ## 2 toyota camry 2.2 1999 4 auto(l4) f 21 27 r midsi… ``` ```r my_cars(mod = "corolla", 2) ``` ``` ## # A tibble: 2 x 11 ## manufacturer model displ year cyl trans drv cty hwy fl class ## <chr> <chr> <dbl> <int> <int> <chr> <chr> <int> <int> <chr> <chr> ## 1 toyota corolla 1.8 1999 4 auto(l3) f 24 30 r compa… ## 2 toyota corolla 1.8 1999 4 auto(l4) f 24 33 r compa… ``` --- ## Order of arguments matter *if unnamed* ```r my_cars(2, "corolla") ``` ``` ## # A tibble: 0 x 11 ## # … with 11 variables: manufacturer <chr>, model <chr>, displ <dbl>, ## # year <int>, cyl <int>, trans <chr>, drv <chr>, cty <int>, hwy <int>, ## # fl <chr>, class <chr> ``` ```r my_cars(2, mod = "corolla") ``` ``` ## # A tibble: 2 x 11 ## manufacturer model displ year cyl trans drv cty hwy fl class ## <chr> <chr> <dbl> <int> <int> <chr> <chr> <int> <int> <chr> <chr> ## 1 toyota corolla 1.8 1999 4 auto(l3) f 24 30 r compa… ## 2 toyota corolla 1.8 1999 4 auto(l4) f 24 33 r compa… ``` ```r my_cars(n = 2, "corolla") ``` ``` ## # A tibble: 2 x 11 ## manufacturer model displ year cyl trans drv cty hwy fl class ## <chr> <chr> <dbl> <int> <int> <chr> <chr> <int> <int> <chr> <chr> ## 1 toyota corolla 1.8 1999 4 auto(l3) f 24 30 r compa… ## 2 toyota corolla 1.8 1999 4 auto(l4) f 24 33 r compa… ``` --- ## Writing functions checklist .pull-left[ Pay attention to: - names of arguments - default argument values - local vs. global objects - return values ] .pull-right[ 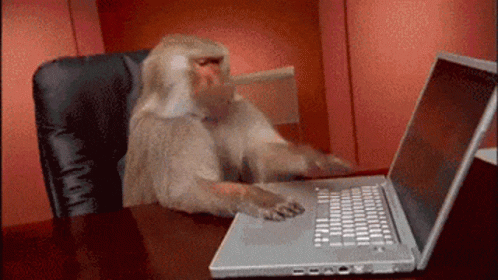 ]